Learning React
Main Concepts
React es una libreria JavaScript
Conceptos basicos del Javascript Modeno
Se definen las variables
let
yconst
en vez devar
.- Porque? Realmente todo es por el scope. JS tiene un block scope.
- Se pueden declarar variables dentro del block (if statement, for loops y eso) sin tener que preocuaparse por sobreescribir alguna variable declarada previamente.
Se puede usar la palabra reservada
class
.- A diferencia de los objetos, uno no necesita poner comas entre la definicion de metodos en una clase, como antes con los prototype.
- En las clases, la palabra clave
this
, en un metodo, puede ser diferente dependiendo de como es llamado.
Las arrow functions representados por
=>
, son regularmente funciones, pero de una forma mas corta. Por ejemplox => x * 2
es el equivalente defunction(x) { return x * 2; }
.- Las arrow function no tiene su propio valor
this
.
- Las arrow function no tiene su propio valor
las variables puedan cambiar (mutables) o no (inmutables). En JavaScript, los array y los objetos son mutables. Para evitar este comportamiento usar el spread operator.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20// En un Array
const stringList = ["Mohan", "Fasale", "Robert"];
const name = "Priya";
const result = [...stringList, name];
console.log(stringList); // ["Mohan","Fasale","Robert"]
console.log(result); // ["Mohan","Fasale","Robert","Priya"]
// En un objeto
const user = {
name: "Mohan",
email: "sample@sss.com",
isEmployee: false,
};
const updatedUser = {
...user,
isEmployee: true,
};
console.log(user); // {name: "Mohan", email: "sample@sss.com", isEmployee: false}
console.log(updatedUser); // {name: "Mohan", email: "sample@sss.com", isEmployee: true}
Closure
Una clausura o closure es una función que guarda referencias del estado adyacente (ámbito léxico o lexical enviroment). En otras palabras, una clausura permite acceder al ámbito de una función exterior desde una función interior.
Lexical scoping
1 | function iniciar() { |
- la funcion
iniciar()
crea una variable local llamadanombre
y una funcion interna llamadamostrarNombre()
. Por ser una funcion interna, esta última solo está disponible dentro del cuerpo deiniciar()
. - Tambien podemos notar que que la funcion
mostrarNombre()
no tiene ninguna variable propia; pero dado que las funciones internas tienen acceso a las funciones externas,mostrarNombre()
puede acceder a la variablenombre
declarada en la funcioniniciar()
. - Al ejecutar la funcion
iniciar()
, esta mostrara la variablenombre
en unalert()
la cual ha sido declarada en la funcion padre.
Closure
Basic Commands
1 | npm init -y |
In package.json
1 | "scripts": { |
Before Hooks
https://reactjs.org/docs/hello-world.html
Render Props
https://reactjs.org/docs/render-props.html
Higher Order Components (HOCs)
https://reactjs.org/docs/higher-order-components.html
After Hooks
Allows for attaching reusable logic to an existing component
Use state and lifecycle methods inside a react functional component
Build 100% of your application with React Functional Components
Three commonly Used React Hooks
useState
Basic state function
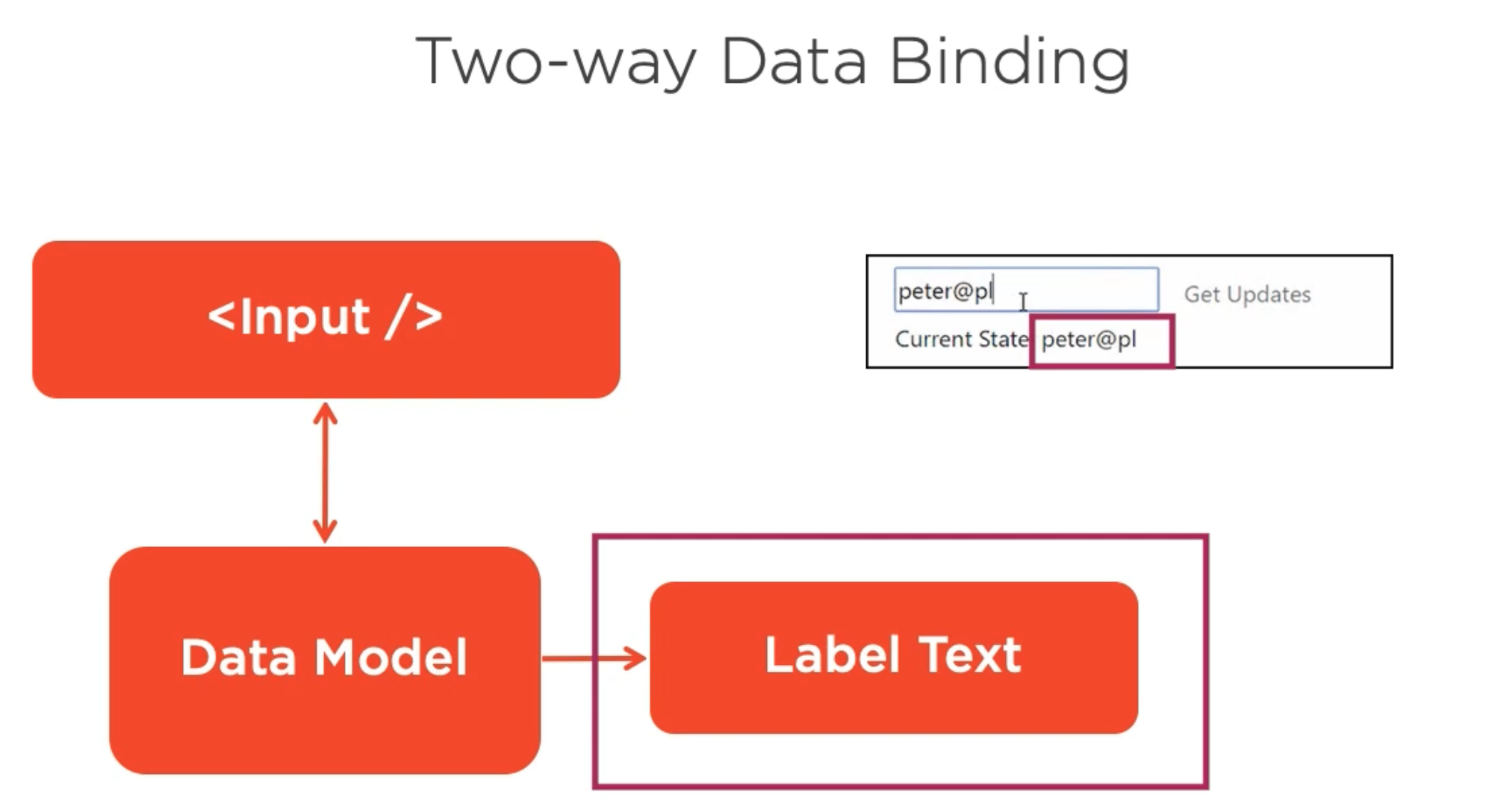
1 | import React, { useState } from "react"; |
useRef
Primarily used to allow access directly to an element in the DOM
1 | import React, { useRef } from "react"; |
useEffect
Using this hook, you tell React that the component needs to do something after render. This function always will be called after performing the DOM updates.
The useEffect
is the equivalent of the lifecycle method componentDidMOunt
, componentDidUpdate
and componentWillUnmount
in a single function.
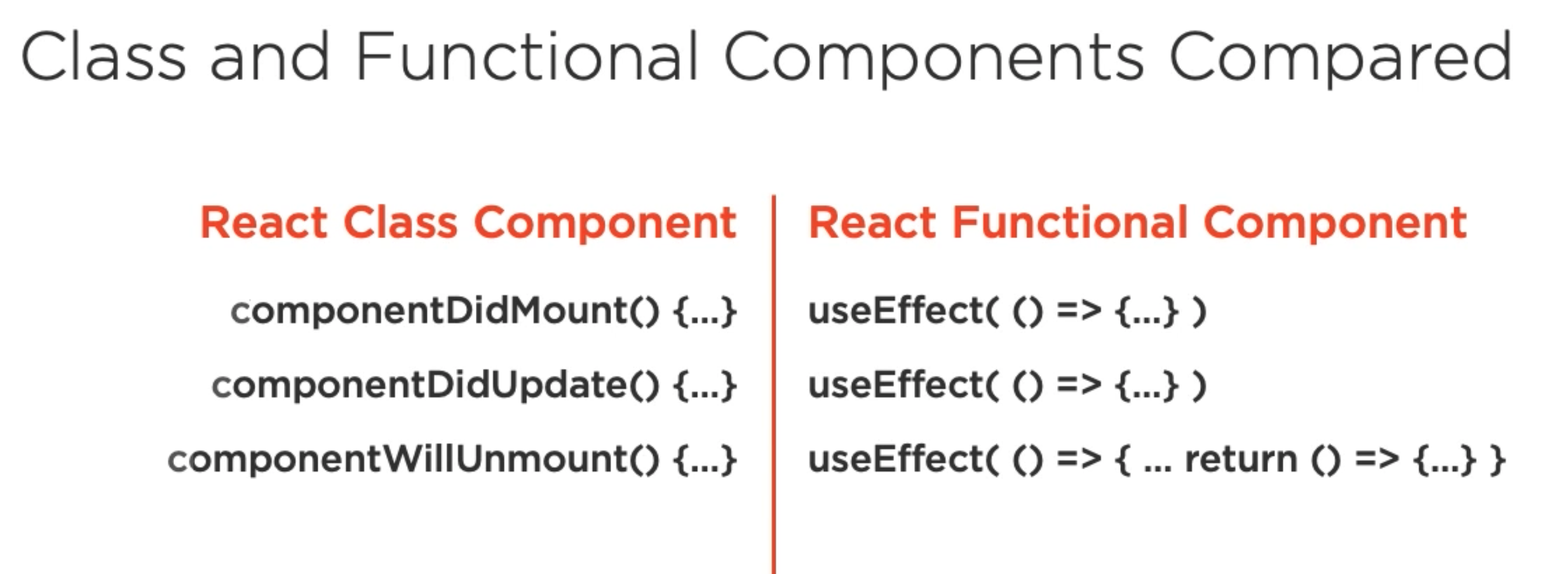
the useEffect
can be used for data fetching, setting up a subscription, and manually changing the DOM in react components.
1 | import React, { useState, useEffect } from "react"; |